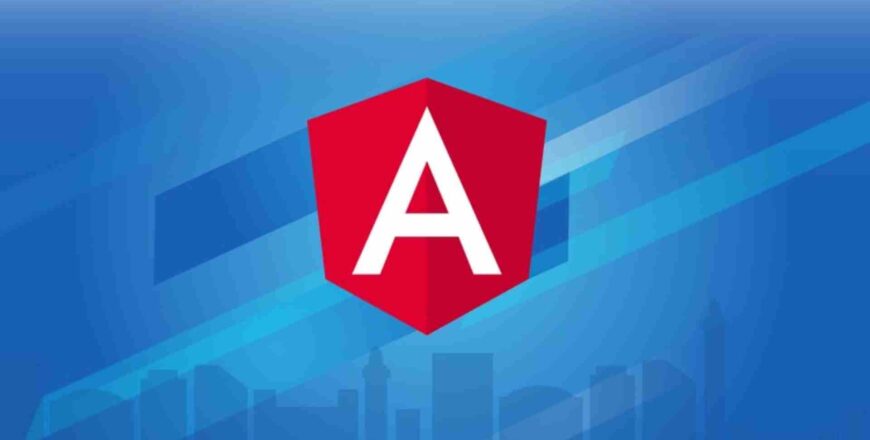
- Descripción
- Currículum
- FAQ
- Reseñas
-
1Course Introduction
Let me introduce you to this course and give a rough outline over what you're going to learn!
-
2What is Angular?
First things first! What is Angular? Why would you want to learn it? This lecture helps answering this question.
-
3Angular vs Angular 2 vs Angular 7
So many Angular versions! What's up with them and which version does this course cover?
-
4CLI Deep Dive & Troubleshooting
Got issues using the CLI, setting up a project or simply want to learn more about it? Check out this lecture.
-
5Project Setup and First App
Enough of the talking, let's create our first Angular project and view our first app in the browser.
-
6Editing the First App
Let's edit our first app!
-
7The Course Structure
How is this course structured? This lecture answers the question and explains what you're going to learn!
-
8How to get the Most out of the Course
Of course you can simply go through all the lectures, but to get the most out of the course, you should consider the advises given in this lecture.
-
9What is TypeScript?
Angular uses TypeScript. What does that mean for you?
-
10A Basic Project Setup using Bootstrap for Styling
A lot of sections of this course will start with a basic setup - this lecture explains how that basic setup is created with the CLI.
-
11Where to find the Course Source Code
Do you get some strange error? Are you stuck? Have a look at the source code of this course.
-
12Module Introduction
Let me introduce you to the module and explain what you're going to learn.
-
13How an Angular App gets Loaded and Started
We saw our first App run in the browser but do you really know how it got there? This lecture answers the question.
-
14Components are Important!
Angular is all about Components! This lectures takes a closer look and explains what Components really are.
-
15Creating a New Component
Thus far, we worked with the AppComponent. Time to change this and create our first own component.
-
16Understanding the Role of AppModule and Component Declaration
Did you recognize that AppModule file? It's super important - this lecture explains what it's about!
-
17Using Custom Components
Now that we learned how to create and register our own components, let's now dive into using them.
-
18Creating Components with the CLI & Nesting Components
We can also use the CLI to create components. This lecture explains how that then works and also how you may nest components.
-
19Working with Component Templates
A Component needs to have a Template. It's an absolute must. This lectures dives deeper into templates.
-
20Working with Component Styles
Whilst a Component is required to have a template, Styles are optional. This lectures explains how you may add styling.
-
21Fully Understanding the Component Selector
The Selector of a Component is important if you want to include it in another template. This lecture explains how that selector actually works and what to watch out for.
-
22Practicing Components
-
23What is Databinding?
Enough about Components for now - let's finally output more dynamic content now. Databinding is super important when it comes to that. This lectures explains what Databinding is.
-
24String Interpolation
One of the simplest forms of Databinding is String Interpolation which allows you to output text in your template. This lecture takes a closer look.
-
25Property Binding
Property Binding is another form of Databinding - also related to outputting content. Learn more about it in this lecture.
-
26Property Binding vs String Interpolation
Since both Property Binding and String Interpolation are related to outputting content, which one should you use? This lecture helps you with that decision!
-
27Event Binding
So far, we only passed data to the template. What if we want to react to (User) Events? Event Binding to the rescue!
-
28Bindable Properties and Events
To which Properties and Events can you bind? This article should be helpful.
-
29Passing and Using Data with Event Binding
When we're talking about Events, we have to consider passing data. This lecture explains how that works.
-
30Important: FormsModule is Required for Two-Way-Binding!
-
31Two-Way-Databinding
You can also combine event and property binding - with Two-Way-Databinding. Learn more about it in this lecture.
-
32Combining all Forms of Databinding
We learned about the different forms of Databinding, let's now combine them!
-
33Practicing Databinding
-
34Understanding Directives
Directives are another important building block in Angular apps. Learn more about it in this lecture.
-
35Using ngIf to Output Data Conditionally
ngIf is one of the built-in directives - it's super helpful if you want to output data dynamically.
-
36Enhancing ngIf with an Else Condition
ngIf is not limited to the usage you learned in the last lecture. Learn how to use it together with an else condition in this lecture.
-
37Styling Elements Dynamically with ngStyle
Want to change some styles dynamically? ngStyle is what you're looking for.
-
38Applying CSS Classes Dynamically with ngClass
Kind of related to the dynamic styling - you can also apply CSS classes dynamically with ngClass.
-
39Outputting Lists with ngFor
What if you wanted to output lists (e.g. an array)? ngFor is there for you.
-
40Practicing Directives
-
41Getting the Index when using ngFor
ngFor also allows you to get the Index of the current iteration - this lecture explains how that works.
-
42Project Introduction
Time to get started with the Course Project.
-
43Planning the App
How should the Angular app we're building look like? Let's plan which features and components we need.
-
44Installing Bootstrap Correctly
This is an important one - make sure to not skip this lecture!
-
45Setting up the Application
Let's get our hands dirty and set the app up.
-
46Creating the Components
Time to create the components we planned to create. Try doing it on your own first!
-
47Using the Components
With the components created in the last lecture, it's now time to use them so that we can see something.
-
48Adding a Navigation Bar
Later in this course, we want to switch pages - setting up a navigation bar sounds like a great idea for that.
-
49Alternative Non-Collapsable Navigation Bar
Our navbar collapses and we don't offer a hamburger menu. Feel free to implement one on your own or change the code as outlined here.
-
50Creating a "Recipe" Model
We're also going to use some data in this project - time to create a model for that data.
-
51Adding Content to the Recipes Components
With the model and the component created, we can now add some content to our component template.
-
52Outputting a List of Recipes with ngFor
In the end, we want to have more than one recipe, so let's prepare our template to output such a list.
-
53Displaying Recipe Details
We also want to display some detailed information about selected recipes, so let's add the appropriate code.
-
54Working on the ShoppingListComponent
We worked on the Recipe Components, let's now do the same for the shopping list.
-
55Creating an "Ingredient" Model
As with the Recipe, we're also going to use some Ingredients in our app - let's add the respective model.
-
56Creating and Outputting the Shopping List
With the model added, we can work on outputting some ingredients.
-
57Adding a Shopping List Edit Section
We also want to be able to add new Ingredients to the Shopping List, so let's add the respective feature.
-
58Wrap Up & Next Steps
Part one of the app is finished. We achieved a lot but a lot of features are also still missing - time to move on and learn more about Angular.
-
59Understanding Angular Error Messages
Things don't always go the way you want them to go. Learn how to read Angular's error messages.
-
60Debugging Code in the Browser Using Sourcemaps
It can be incredibly useful to debug your app in the browser - learn more in this lecture.
-
61Using Augury to Dive into Angular Apps
Augury is a nice plugin you can use to dive deeply into your app in the browser.
-
62Module Introduction
We already learned some things about components but now it's time to dive deeper into them!
-
63Splitting Apps into Components
This lecture explains how you may split an existing app into multiple new components.
-
64Property & Event Binding Overview
You already learned about property and event binding - but you didn't learn everything about it. Time to do so now.
-
65Binding to Custom Properties
You're not limited to binding to built-in properties. Indeed, binding to custom property is a key feature of Angular apps. Time to learn more about it.
-
66Assigning an Alias to Custom Properties
Sometimes, you want to use a different property name outside of a component than inside of it. This lecture explains how to do that.
-
67Binding to Custom Events
As with property binding, you can also bind to custom events.
-
68Assigning an Alias to Custom Events
You may also assign an alias to your custom events.
-
69Custom Property and Event Binding Summary
Let me summarize the things you learned about property and event binding.
-
70Understanding View Encapsulation
Angular allows you to apply different styles to different components - this lecture explains how that works.
-
71More on View Encapsulation
Let's dive deeper into View Encapsulation.
-
72Using Local References in Templates
Sometimes, you want to get access to some of your HTML elements. Local references allow you to do just that.
-
73Getting Access to the Template & DOM with @ViewChild
You got the local references in the templates, but you can also access your elements directly from the TypeScript file - this lecture explains how that works.
-
74Projecting Content into Components with ng-content
Want to pass structured content (e.g. HTML code) into another component? Learn more about it in this lecture.
-
75Understanding the Component Lifecycle
Components follow a certain lifecycle - this lecture dives deeper into this topic.
-
76Seeing Lifecycle Hooks in Action
Let's see those lifecycle hooks in action.
-
77Lifecycle Hooks and Template Access
How can we access template elements in different lifecycle hooks? Let's find out ...
-
78Getting Access to ng-content with @ContentChild
You may also get access to the content projected into a component - let's also see how that works in lifecycle hooks.
-
79Wrap Up
Let me wrap up this section about Components & Databinding
-
80Practicing Property & Event Binding and View Encapsulation
-
81Introduction
Now that we learned how to pass data to components, let's enhance our app.
-
82Adding Navigation with Event Binding and ngIf
Let's use the new features to add a first version of our app navigation - using custom events and ngIf.
-
83Passing Recipe Data with Property Binding
We can not only listen to our own events, we can also pass data to components now, so let's do so.
-
84Passing Data with Event and Property Binding (Combined)
Let's build a more complex chain of custom property and event binding to pass data around.
-
85Make sure you have FormsModule added!
-
86Allowing the User to Add Ingredients to the Shopping List
The user should also be able to add ingredients to the shopping list, so let's add such a feature.